11. MOEA#
import warnings; warnings.simplefilter('ignore')
!wget https://raw.githubusercontent.com/cfteach/modules/master/detector2.py
!pip install pymoo > /dev/null 2>&1
!pip install plotly > /dev/null 2>&1
!pip install ipyvolume > /dev/null 2>&1
!pip install altair > /dev/null 2>&1
--2024-07-10 15:44:46-- https://raw.githubusercontent.com/cfteach/modules/master/detector2.py
Resolving raw.githubusercontent.com (raw.githubusercontent.com)... 185.199.108.133, 185.199.111.133, 185.199.110.133, ...
Connecting to raw.githubusercontent.com (raw.githubusercontent.com)|185.199.108.133|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 7976 (7.8K) [text/plain]
Saving to: ‘detector2.py.5’
detector2.py.5 100%[===================>] 7.79K --.-KB/s in 0.005s
2024-07-10 15:44:46 (1.62 MB/s) - ‘detector2.py.5’ saved [7976/7976]
%load_ext autoreload
%autoreload 2
import os
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
#import AI4NP_detector_opt.sol2.detector2 as detector2
import detector2
from pymoo.algorithms.moo.nsga2 import NSGA2
from pymoo.core.problem import Problem
from pymoo.optimize import minimize
from pymoo.visualization.scatter import Scatter
from pymoo.util.display.column import Column
from pymoo.util.display.output import Output
from pymoo.util.nds.non_dominated_sorting import NonDominatedSorting
The autoreload extension is already loaded. To reload it, use:
%reload_ext autoreload
11.1. Create detector geometry and simulate tracks#
The module detector creates a simple 2D geometry of a wire based tracker made by 4 planes.
The adjustable parameters are the radius of each wire, the pitch (along the y axis), and the shift along y and z of a plane with respect to the previous one.
A total of 8 parameters can be tuned.
The goal of this toy model, is to tune the detector design so to optimize the efficiency (fraction of tracks which are detected) as well as the cost for its realization. As a proxy for the cost, we use the material/volume (the surface in 2D) of the detector. For a track to be detetected, in the efficiency definition we require at least two wires hit by the track.
So we want to maximize the efficiency (defined in detector.py) and minimize the cost.
11.1.1. LIST OF PARAMETERS#
(baseline values)
R = .5 [cm]
pitch = 4.0 [cm]
y1 = 0.0, y2 = 0.0, y3 = 0.0, z1 = 2.0, z2 = 4.0, z3 = 6.0 [cm]
# CONSTANT PARAMETERS
#------ define mother region ------#
y_min=-10.1
y_max=10.1
N_tracks = 1000
print("::::: BASELINE PARAMETERS :::::")
R = 0.5
pitch = 4.0
y1 = 2.0
y2 = 1.0
y3 = 0.0
z1 = 2.0
z2 = 4.0
z3 = 6.0
print("R, pitch, y1, y2, y3, z1, z2, z3: ", R, pitch, y1, y2, y3, z1, z2, z3,"\n")
#------------- GEOMETRY ---------------#
print(":::: INITIAL GEOMETRY ::::")
tr = detector2.Tracker(R, pitch, y1, y2, y3, z1, z2, z3)
Z, Y = tr.create_geometry()
num_wires = detector2.calculate_wires(Y, y_min, y_max)
volume = detector2.wires_volume(Y, y_min, y_max,R)
detector2.geometry_display(Z, Y, R, y_min=y_min, y_max=y_max,block=False,pause=5) #5
print("# of wires: ", num_wires, ", volume: ", volume)
#------------- TRACK GENERATION -----------#
print(":::: TRACK GENERATION ::::")
t = detector2.Tracks(b_min=y_min, b_max=y_max, alpha_mean=0, alpha_std=0.3)
tracks = t.generate(N_tracks)
detector2.geometry_display(Z, Y, R, y_min=y_min, y_max=y_max,block=False, pause=-1)
detector2.tracks_display(tracks, Z,block=False,pause=-1)
#a track is detected if at least two wires have been hit
score = detector2.get_score(Z, Y, tracks, R)
frac_detected = score[0]
resolution = score[1]
print("fraction of tracks detected: ",frac_detected)
print("resolution: ",resolution)
::::: BASELINE PARAMETERS :::::
R, pitch, y1, y2, y3, z1, z2, z3: 0.5 4.0 2.0 1.0 0.0 2.0 4.0 6.0
:::: INITIAL GEOMETRY ::::
# of wires: 21 , volume: 65.94
:::: TRACK GENERATION ::::
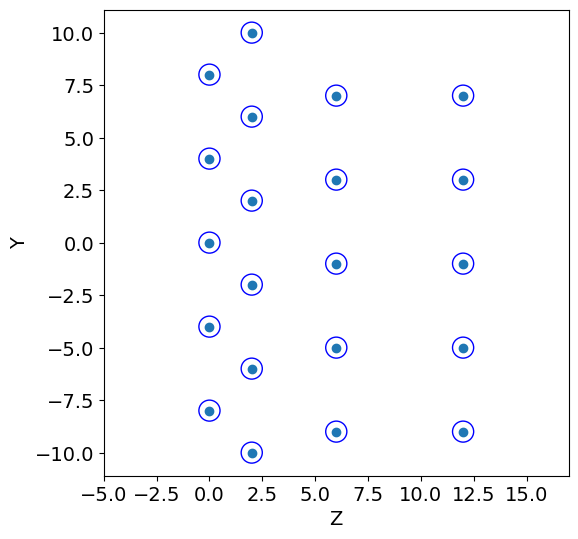
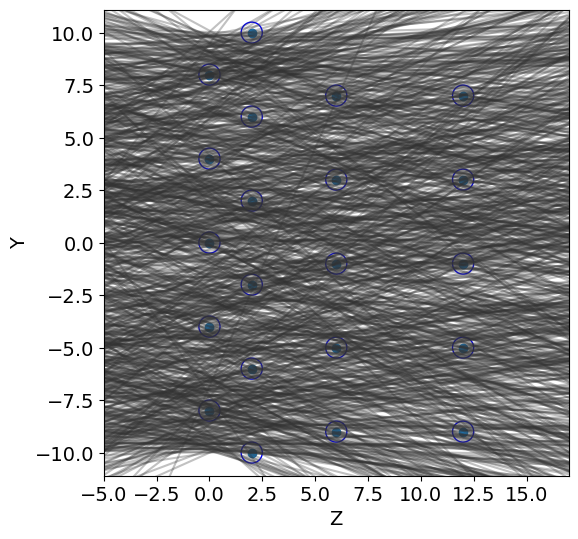
fraction of tracks detected: 0.259
resolution: 0.2405574316639721
11.2. Define Objectives#
Defines a class for the objectives of the problem that can be used in the MOO.
# The objectives are computed by setting up the geometry and
# computing the values of the objectives. Mimicing a G4 type simulation
class objectives():
def __init__(self,tracks,y_min,y_max):
self.tracks = tracks
self.y_min = y_min
self.y_max = y_max
def wrapper_geometry(fun):
def inner(self):
R, pitch, y1, y2, y3, z1, z2, z3 = self.X
self.geometry(R, pitch, y1, y2, y3, z1, z2, z3)
return fun(self)
return inner
def update_tracks(self, new_tracks):
self.tracks = new_tracks
def update_design_point(self,X):
self.X = X
def geometry(self,R, pitch, y1, y2, y3, z1, z2, z3):
tr = detector2.Tracker(R, pitch, y1, y2, y3, z1, z2, z3)
self.R = R
self.Z, self.Y = tr.create_geometry()
@wrapper_geometry
def calc_score(self):
res = detector2.get_score(self.Z, self.Y, self.tracks, self.R)
assert res[0] >= 0 and res[1] >= 0,"Fraction or Resolution negative."
return res
def get_score(self,X):
R, pitch, y1, y2, y3, z1, z2, z3 = X
self.geometry(R, pitch, y1, y2, y3, z1, z2, z3)
res = detector2.get_score(self.Z, self.Y, self.tracks, self.R)
return res
def get_volume(self):
volume = detector2.wires_volume(self.Y, self.y_min, self.y_max,self.R)
return volume
res = objectives(tracks,y_min,y_max)
#res.geometry(R, pitch, y1, y2, y3, z1, z2, z3)
X = R, pitch, y1, y2, y3, z1, z2, z3
#fscore = res.get_score(X)
res.update_design_point(X)
fscore = res.calc_score()[0]
fvolume = res.get_volume()
print("...check: ", fvolume, fscore)
...check: 65.94 0.259
11.3. Multi-Objective Optimization#
We will be using pymoo (https://pymoo.org/getting_started.html).
In the constructor method we specify number of variables N, objectives M, and constraint functions, as well as the lower and upper boundaries of each variable. In our toy model, these boundaries are taken in such a way that all solutions are feasible and no constraint function is needed. You can try to change this and introduce some constraint.
The _evaluate method takes a one-dimensional NumPy array x number of entries equal to n_var. This behavior is enabled by setting elementwise_evaluation=True while calling the super() method.
Notice that every function is minimized. Our efficiency is defined as an tracking inefficiency = 1 - efficiency
We add the resolution as a third objective. The average residual of the track hit from the wire centre is used as a proxy for the resolution for this toy-model
from pymoo.core.problem import ElementwiseProblem
class MyProblem(ElementwiseProblem):
#--------- vectorized ---------#
def __init__(self, n_var=8, n_obj=2, n_constr=0,
xl=np.array([0.5,2.5,0.,0.,0.,2.,2.,2.]),
xu=np.array([1.0,5.0,4.,4.,4.,10.,10.,10.])
):
super().__init__(n_var=n_var, n_obj=n_obj, n_constr=n_constr, xl=xl, xu=xu)
def _evaluate(self, x, out, *args, **kwargs):
f1 = 1.- res.get_score(x)[0] # Ineffiency
f2 = res.get_volume() # volume
#f3 = res.get_score(x)[1] #resolution
out["F"] = [f1, f2]#, f3]
11.4. Creation of Problem and choice of optimization algorithm.#
We will use NSGA-II, as explained in the lectures. You can decide the population size and the number of offsprings, based on what we discussed.
Pymoo offers different algorithms that can be used which are highly customizable and can be easily extended. https://pymoo.org/algorithms/index.html
Before dealing with a problem, it’s useful to compare with a list of test problems reported in https://pymoo.org/problems/index.html, where different scenarios in terms of Variables, Objectives, Constraints are described.
n_var = 8 # number of design variables
n_obj = 2 # number of objectives
n_constr = 0 # number of constraints
xl = np.array([0.5,2.5,0.,0.,0.,2.,2.,2.]) # lower bounds of the problem, pitch, diameter, y1, y2, y3, z1, z2, z3
xu = np.array([1.0,5.0,4.,4.,4.,10.,10.,10.]) # upper bounds of the problem, pitch, diameter, y1, y2, y3, z1, z2, z3
pop_size = 40
n_offsprings=10
problem = MyProblem(n_var=n_var, n_obj=n_obj, n_constr=n_constr, xl=xl, xu=xu)
from pymoo.algorithms.moo.nsga2 import NSGA2
from pymoo.operators.crossover.sbx import SBX
from pymoo.operators.mutation.pm import PM
from pymoo.operators.sampling.rnd import FloatRandomSampling
from pymoo.termination import get_termination
from pymoo.termination.xtol import DesignSpaceTermination
from pymoo.termination.robust import RobustTermination
# check out other sampling methods here https://pymoo.org/operators/sampling.html
# check out other crossover methods here https://pymoo.org/operators/crossover.html
algorithm = NSGA2(pop_size=pop_size,n_offsprings=10)
crossover = SBX(prob=1.0, eta=1)
mutation = PM(eta=1)
termination = RobustTermination(DesignSpaceTermination(tol=0.01), period=20)
res = minimize(problem,
algorithm,
crossover = crossover,
mutation = mutation,
termination = termination,
verbose=True,
seed=1,
save_history=True # Saves all of the history of the algorithm
)
==========================================================
n_gen | n_eval | n_nds | eps | indicator
==========================================================
1 | 40 | 11 | - | -
2 | 50 | 13 | 0.0871021776 | ideal
3 | 60 | 16 | 0.1372832370 | ideal
4 | 70 | 17 | 0.0034332054 | f
5 | 80 | 18 | 0.0093329792 | f
6 | 90 | 19 | 0.0156472262 | ideal
7 | 100 | 20 | 0.0021744597 | f
8 | 110 | 21 | 0.0036942297 | f
9 | 120 | 21 | 0.0029114566 | f
10 | 130 | 21 | 0.0051548483 | f
11 | 140 | 25 | 0.0054760242 | f
12 | 150 | 28 | 0.0202898551 | nadir
13 | 160 | 28 | 0.0063842991 | f
14 | 170 | 28 | 0.0023386570 | f
15 | 180 | 30 | 0.0033706084 | f
16 | 190 | 35 | 0.0368487929 | ideal
17 | 200 | 38 | 0.0014237101 | f
18 | 210 | 39 | 0.0396301189 | nadir
19 | 220 | 36 | 0.0018665763 | f
20 | 230 | 37 | 0.0044082882 | f
21 | 240 | 38 | 0.0181582361 | nadir
22 | 250 | 37 | 0.0038759690 | ideal
23 | 260 | 40 | 0.0011090398 | f
24 | 270 | 40 | 0.0028585273 | f
25 | 280 | 40 | 0.0018497284 | f
26 | 290 | 40 | 0.0170827858 | nadir
27 | 300 | 40 | 0.0016562690 | f
28 | 310 | 38 | 0.0039496464 | f
29 | 320 | 39 | 0.0563041784 | nadir
30 | 330 | 38 | 0.0011071712 | f
31 | 340 | 39 | 0.0034587737 | f
32 | 350 | 36 | 0.0039215686 | ideal
33 | 360 | 39 | 0.0022945863 | f
34 | 370 | 40 | 0.0132450331 | nadir
35 | 380 | 40 | 0.0026420079 | ideal
36 | 390 | 40 | 0.0012435099 | f
37 | 400 | 40 | 0.0017644339 | f
38 | 410 | 37 | 0.0022415008 | f
39 | 420 | 37 | 0.0037959332 | f
40 | 430 | 37 | 0.000000E+00 | f
41 | 440 | 40 | 0.0022902098 | f
42 | 450 | 40 | 0.0023986911 | f
43 | 460 | 40 | 0.0034726314 | f
44 | 470 | 40 | 0.0008651538 | f
45 | 480 | 40 | 0.0018117797 | f
46 | 490 | 40 | 0.0030422684 | f
47 | 500 | 40 | 0.0007731441 | f
48 | 510 | 40 | 0.0007731441 | f
49 | 520 | 39 | 0.0299319728 | nadir
50 | 530 | 40 | 0.0019056427 | f
51 | 540 | 40 | 0.0027704680 | f
52 | 550 | 40 | 0.0147453083 | ideal
53 | 560 | 39 | 0.0019503080 | f
54 | 570 | 40 | 0.0026728838 | f
55 | 580 | 40 | 0.0011651337 | f
56 | 590 | 40 | 0.0011651337 | f
57 | 600 | 40 | 0.0011651337 | f
58 | 610 | 39 | 0.0019867235 | f
59 | 620 | 40 | 0.0023424366 | f
60 | 630 | 40 | 0.0106100796 | nadir
61 | 640 | 40 | 0.0001989390 | f
62 | 650 | 40 | 0.0009440195 | f
63 | 660 | 38 | 0.0015318531 | f
64 | 670 | 38 | 0.0016970421 | f
65 | 680 | 39 | 0.0021987770 | f
66 | 690 | 39 | 0.0021987770 | f
67 | 700 | 40 | 0.0025752802 | f
68 | 710 | 40 | 0.0003844496 | f
69 | 720 | 40 | 0.0004176061 | f
70 | 730 | 40 | 0.0016500963 | f
71 | 740 | 40 | 0.0026396206 | f
72 | 750 | 40 | 0.0011177721 | f
73 | 760 | 40 | 0.0015715889 | f
74 | 770 | 40 | 0.0024569502 | f
75 | 780 | 40 | 0.0024569502 | f
76 | 790 | 40 | 0.0030537672 | f
77 | 800 | 40 | 0.0001854089 | f
78 | 810 | 39 | 0.0010895433 | f
79 | 820 | 39 | 0.0010895433 | f
80 | 830 | 39 | 0.0010895433 | f
81 | 840 | 39 | 0.0010895433 | f
82 | 850 | 40 | 0.0015089697 | f
83 | 860 | 40 | 0.0036490451 | f
84 | 870 | 40 | 0.0007378275 | f
85 | 880 | 40 | 0.0008548538 | f
86 | 890 | 40 | 0.0024318595 | f
87 | 900 | 40 | 0.0065876153 | nadir
88 | 910 | 40 | 0.0008741920 | f
89 | 920 | 40 | 0.0016074147 | f
90 | 930 | 40 | 0.0021846088 | f
91 | 940 | 40 | 0.0026614711 | f
92 | 950 | 40 | 0.0008959544 | f
93 | 960 | 40 | 0.0023483533 | f
94 | 970 | 40 | 0.0027061989 | f
95 | 980 | 40 | 0.0008279308 | f
96 | 990 | 40 | 0.0008279308 | f
97 | 1000 | 40 | 0.0016148320 | f
98 | 1010 | 40 | 0.0031454717 | f
99 | 1020 | 40 | 0.0147058824 | nadir
100 | 1030 | 40 | 0.0003875909 | f
101 | 1040 | 40 | 0.0023512445 | f
102 | 1050 | 40 | 0.0023745841 | f
103 | 1060 | 40 | 0.0053191489 | nadir
104 | 1070 | 40 | 0.0011538971 | f
105 | 1080 | 40 | 0.0014355957 | f
106 | 1090 | 40 | 0.0024817779 | f
107 | 1100 | 40 | 0.0034383600 | f
108 | 1110 | 40 | 0.0818070818 | nadir
109 | 1120 | 40 | 0.0301886792 | nadir
110 | 1130 | 40 | 0.0000628931 | f
111 | 1140 | 40 | 0.0004492863 | f
112 | 1150 | 40 | 0.0008391066 | f
113 | 1160 | 40 | 0.0009019997 | f
114 | 1170 | 40 | 0.0014170529 | f
115 | 1180 | 40 | 0.0014170529 | f
116 | 1190 | 40 | 0.0026094245 | f
117 | 1200 | 40 | 0.0007724952 | f
118 | 1210 | 40 | 0.0007724952 | f
119 | 1220 | 40 | 0.0016864726 | f
120 | 1230 | 40 | 0.0023964510 | f
121 | 1240 | 40 | 0.0029834894 | f
122 | 1250 | 40 | 0.000000E+00 | f
123 | 1260 | 40 | 0.0001459303 | f
124 | 1270 | 40 | 0.0001757902 | f
125 | 1280 | 40 | 0.0006486684 | f
126 | 1290 | 40 | 0.0009010120 | f
127 | 1300 | 40 | 0.0011300096 | f
128 | 1310 | 40 | 0.0012559542 | f
129 | 1320 | 40 | 0.0015442368 | f
130 | 1330 | 40 | 0.0019409070 | f
131 | 1340 | 40 | 0.0023903297 | f
132 | 1350 | 40 | 0.0023903297 | f
133 | 1360 | 40 | 0.0088945362 | nadir
134 | 1370 | 40 | 0.000000E+00 | f
135 | 1380 | 40 | 0.0037974684 | ideal
136 | 1390 | 40 | 0.0010749928 | f
137 | 1400 | 40 | 0.0010749928 | f
138 | 1410 | 40 | 0.0010749928 | f
139 | 1420 | 40 | 0.0010749928 | f
140 | 1430 | 40 | 0.0076530612 | nadir
141 | 1440 | 40 | 0.0002057668 | f
142 | 1450 | 40 | 0.0002057668 | f
143 | 1460 | 40 | 0.0007747530 | f
144 | 1470 | 40 | 0.0014425900 | f
145 | 1480 | 40 | 0.0017113956 | f
146 | 1490 | 40 | 0.0019666061 | f
147 | 1500 | 40 | 0.0025006999 | f
148 | 1510 | 40 | 0.0000318878 | f
149 | 1520 | 40 | 0.0000318878 | f
150 | 1530 | 40 | 0.0002869922 | f
151 | 1540 | 40 | 0.0005743462 | f
152 | 1550 | 40 | 0.0008473664 | f
153 | 1560 | 40 | 0.0064184852 | nadir
154 | 1570 | 40 | 0.0013543801 | f
155 | 1580 | 40 | 0.0013261611 | f
156 | 1590 | 40 | 0.0018643668 | f
157 | 1600 | 40 | 0.0019533794 | f
158 | 1610 | 40 | 0.0018541345 | f
159 | 1620 | 40 | 0.0018541345 | f
160 | 1630 | 40 | 0.0018541345 | f
161 | 1640 | 40 | 0.0019509633 | f
162 | 1650 | 40 | 0.0019297539 | f
163 | 1660 | 40 | 0.0019297539 | f
164 | 1670 | 40 | 0.0019297539 | f
165 | 1680 | 40 | 0.0026110436 | f
166 | 1690 | 40 | 0.000000E+00 | f
167 | 1700 | 40 | 0.0001316125 | f
168 | 1710 | 40 | 0.0003883519 | f
169 | 1720 | 40 | 0.0005372479 | f
170 | 1730 | 40 | 0.0009539918 | f
171 | 1740 | 40 | 0.0009539918 | f
172 | 1750 | 40 | 0.0009539918 | f
173 | 1760 | 40 | 0.0011621699 | f
174 | 1770 | 40 | 0.0013084637 | f
175 | 1780 | 40 | 0.0015401736 | f
176 | 1790 | 40 | 0.0019950562 | f
177 | 1800 | 40 | 0.0027153134 | f
178 | 1810 | 40 | 0.0001363723 | f
179 | 1820 | 40 | 0.0010340368 | f
180 | 1830 | 40 | 0.0012268389 | f
181 | 1840 | 40 | 0.0012268389 | f
182 | 1850 | 40 | 0.0014794106 | f
183 | 1860 | 40 | 0.0014794106 | f
184 | 1870 | 40 | 0.0017941213 | f
185 | 1880 | 40 | 0.0018905223 | f
186 | 1890 | 40 | 0.0018905223 | f
187 | 1900 | 40 | 0.0018955442 | f
188 | 1910 | 40 | 0.0018955442 | f
189 | 1920 | 40 | 0.0025461279 | f
190 | 1930 | 40 | 0.0004203387 | f
191 | 1940 | 40 | 0.0007652219 | f
192 | 1950 | 40 | 0.0005723906 | f
193 | 1960 | 39 | 0.0025641026 | ideal
194 | 1970 | 40 | 0.0006752266 | f
195 | 1980 | 40 | 0.0010848300 | f
196 | 1990 | 40 | 0.0013804698 | f
197 | 2000 | 40 | 0.0014766237 | f
198 | 2010 | 40 | 0.0014766237 | f
199 | 2020 | 40 | 0.0019377145 | f
200 | 2030 | 40 | 0.0019377145 | f
201 | 2040 | 40 | 0.0019377145 | f
202 | 2050 | 40 | 0.0024219295 | f
203 | 2060 | 40 | 0.0033194129 | f
204 | 2070 | 40 | 0.0005980229 | f
205 | 2080 | 40 | 0.0024478628 | f
206 | 2090 | 40 | 0.0024478628 | f
207 | 2100 | 40 | 0.0028324782 | f
208 | 2110 | 40 | 0.0001295843 | f
209 | 2120 | 40 | 0.0005855724 | f
210 | 2130 | 40 | 0.0012914828 | f
211 | 2140 | 40 | 0.0018422310 | f
212 | 2150 | 40 | 0.0023550516 | f
213 | 2160 | 40 | 0.0024150474 | f
214 | 2170 | 40 | 0.0126582278 | nadir
215 | 2180 | 40 | 0.0000321095 | f
216 | 2190 | 40 | 0.0000321095 | f
217 | 2200 | 40 | 0.0000321095 | f
218 | 2210 | 40 | 0.0007162649 | f
219 | 2220 | 39 | 0.0180412371 | nadir
220 | 2230 | 39 | 0.0000330426 | f
221 | 2240 | 40 | 0.0005478804 | f
222 | 2250 | 40 | 0.0005154639 | f
223 | 2260 | 40 | 0.0006443299 | f
224 | 2270 | 40 | 0.0010588172 | f
225 | 2280 | 40 | 0.0010588172 | f
226 | 2290 | 40 | 0.0064850843 | nadir
227 | 2300 | 40 | 0.0131406045 | nadir
228 | 2310 | 40 | 0.000000E+00 | f
229 | 2320 | 40 | 0.000000E+00 | f
230 | 2330 | 40 | 0.0009527561 | f
231 | 2340 | 40 | 0.0013798258 | f
232 | 2350 | 40 | 0.0015361295 | f
233 | 2360 | 40 | 0.0023671360 | f
234 | 2370 | 40 | 0.0028731112 | f
235 | 2380 | 40 | 0.0000657362 | f
236 | 2390 | 40 | 0.0052287582 | nadir
237 | 2400 | 40 | 0.000000E+00 | f
238 | 2410 | 40 | 0.000000E+00 | f
239 | 2420 | 40 | 0.0000691295 | f
240 | 2430 | 40 | 0.0007454628 | f
241 | 2440 | 40 | 0.0007781425 | f
242 | 2450 | 40 | 0.0009803137 | f
243 | 2460 | 40 | 0.0017004670 | f
244 | 2470 | 40 | 0.0017004670 | f
245 | 2480 | 40 | 0.0024194212 | f
246 | 2490 | 40 | 0.0024194212 | f
247 | 2500 | 40 | 0.0032880852 | f
248 | 2510 | 40 | 0.0002287582 | f
249 | 2520 | 40 | 0.0002287582 | f
250 | 2530 | 40 | 0.0006593793 | f
251 | 2540 | 40 | 0.0145888594 | nadir
252 | 2550 | 40 | 0.000000E+00 | f
253 | 2560 | 40 | 0.0008636570 | f
254 | 2570 | 40 | 0.0011289090 | f
255 | 2580 | 40 | 0.0011439341 | f
256 | 2590 | 40 | 0.0011439341 | f
257 | 2600 | 40 | 0.0014730806 | f
258 | 2610 | 38 | 0.0022041319 | f
259 | 2620 | 39 | 0.0029544128 | f
260 | 2630 | 38 | 0.0013625597 | f
261 | 2640 | 39 | 0.0012274446 | f
262 | 2650 | 40 | 0.0021733830 | f
263 | 2660 | 40 | 0.0035578294 | f
264 | 2670 | 40 | 0.0005959703 | f
265 | 2680 | 38 | 0.0009403720 | f
266 | 2690 | 40 | 0.0016824175 | f
267 | 2700 | 40 | 0.0020933679 | f
268 | 2710 | 40 | 0.0020933679 | f
269 | 2720 | 40 | 0.0020933679 | f
270 | 2730 | 40 | 0.0020933679 | f
271 | 2740 | 40 | 0.0024330052 | f
272 | 2750 | 40 | 0.0027415375 | f
273 | 2760 | 40 | 0.0005445573 | f
274 | 2770 | 40 | 0.0011115312 | f
275 | 2780 | 40 | 0.0011115312 | f
276 | 2790 | 40 | 0.0013895499 | f
277 | 2800 | 40 | 0.0013895499 | f
278 | 2810 | 40 | 0.0194805195 | nadir
279 | 2820 | 40 | 0.0010264558 | f
280 | 2830 | 40 | 0.0011656520 | f
281 | 2840 | 40 | 0.0019022902 | f
282 | 2850 | 40 | 0.0024248074 | f
283 | 2860 | 40 | 0.0024572952 | f
284 | 2870 | 40 | 0.0039516563 | f
285 | 2880 | 40 | 0.0001948052 | f
286 | 2890 | 40 | 0.0012977720 | f
287 | 2900 | 40 | 0.0012977720 | f
288 | 2910 | 40 | 0.0017927314 | f
289 | 2920 | 40 | 0.0017927314 | f
290 | 2930 | 40 | 0.0020849392 | f
291 | 2940 | 40 | 0.0022698513 | f
292 | 2950 | 40 | 0.0029947970 | f
293 | 2960 | 40 | 0.0000324675 | f
294 | 2970 | 40 | 0.0003897097 | f
295 | 2980 | 40 | 0.0003897097 | f
296 | 2990 | 40 | 0.0010242752 | f
297 | 3000 | 40 | 0.0010567428 | f
298 | 3010 | 40 | 0.0013706067 | f
299 | 3020 | 40 | 0.0014940275 | f
300 | 3030 | 40 | 0.0020131212 | f
301 | 3040 | 40 | 0.0021105238 | f
302 | 3050 | 40 | 0.0027378644 | f
303 | 3060 | 40 | 0.0006216502 | f
304 | 3070 | 40 | 0.0065359477 | nadir
305 | 3080 | 40 | 0.000000E+00 | f
306 | 3090 | 40 | 0.0002681625 | f
307 | 3100 | 40 | 0.0008237186 | f
308 | 3110 | 40 | 0.0008237186 | f
309 | 3120 | 40 | 0.0028966309 | f
310 | 3130 | 40 | 0.000000E+00 | f
311 | 3140 | 40 | 0.0006894872 | f
312 | 3150 | 40 | 0.0006872605 | f
313 | 3160 | 40 | 0.0006872605 | f
314 | 3170 | 40 | 0.0006872605 | f
315 | 3180 | 40 | 0.0006872605 | f
316 | 3190 | 40 | 0.0014955631 | f
317 | 3200 | 40 | 0.0016739164 | f
318 | 3210 | 39 | 0.0014995080 | f
319 | 3220 | 38 | 0.0015733685 | f
320 | 3230 | 38 | 0.0023992530 | f
321 | 3240 | 40 | 0.0035843868 | f
322 | 3250 | 40 | 0.0000980392 | f
323 | 3260 | 40 | 0.0003435413 | f
324 | 3270 | 40 | 0.0008013922 | f
325 | 3280 | 40 | 0.0008994314 | f
326 | 3290 | 40 | 0.0008994314 | f
327 | 3300 | 40 | 0.0011096303 | f
328 | 3310 | 40 | 0.0016325061 | f
329 | 3320 | 40 | 0.0016325061 | f
330 | 3330 | 40 | 0.0020852041 | f
331 | 3340 | 40 | 0.0020852041 | f
332 | 3350 | 40 | 0.0020852041 | f
333 | 3360 | 40 | 0.0025054097 | f
334 | 3370 | 40 | 0.000000E+00 | f
335 | 3380 | 40 | 0.000000E+00 | f
336 | 3390 | 40 | 0.000000E+00 | f
337 | 3400 | 40 | 0.000000E+00 | f
338 | 3410 | 40 | 0.000000E+00 | f
339 | 3420 | 40 | 0.0008306514 | f
340 | 3430 | 40 | 0.0008306514 | f
341 | 3440 | 40 | 0.0014647355 | f
342 | 3450 | 40 | 0.0015627747 | f
343 | 3460 | 40 | 0.0016281342 | f
344 | 3470 | 40 | 0.0017588532 | f
345 | 3480 | 40 | 0.0024187602 | f
346 | 3490 | 40 | 0.0026824641 | f
347 | 3500 | 40 | 0.0000326797 | f
348 | 3510 | 40 | 0.0002837271 | f
349 | 3520 | 40 | 0.0002837271 | f
350 | 3530 | 40 | 0.0008429955 | f
351 | 3540 | 40 | 0.0009445374 | f
352 | 3550 | 40 | 0.0009445374 | f
353 | 3560 | 40 | 0.0020213458 | f
354 | 3570 | 40 | 0.0018811487 | f
355 | 3580 | 40 | 0.0039370079 | nadir
356 | 3590 | 40 | 0.0000656168 | f
357 | 3600 | 40 | 0.0000656168 | f
358 | 3610 | 40 | 0.0000656168 | f
359 | 3620 | 40 | 0.0000656168 | f
360 | 3630 | 40 | 0.0005577428 | f
361 | 3640 | 40 | 0.0007819121 | f
362 | 3650 | 40 | 0.0007819121 | f
363 | 3660 | 39 | 0.0026178010 | ideal
364 | 3670 | 39 | 0.000000E+00 | f
365 | 3680 | 40 | 0.0004702676 | f
366 | 3690 | 40 | 0.0010019335 | f
367 | 3700 | 40 | 0.0010577693 | f
368 | 3710 | 40 | 0.0011232144 | f
369 | 3720 | 40 | 0.0011232144 | f
370 | 3730 | 40 | 0.0012868293 | f
371 | 3740 | 40 | 0.0012868293 | f
372 | 3750 | 40 | 0.0013522744 | f
373 | 3760 | 40 | 0.0026109661 | nadir
374 | 3770 | 40 | 0.0001305483 | f
375 | 3780 | 40 | 0.0001631854 | f
376 | 3790 | 40 | 0.0005735239 | f
377 | 3800 | 40 | 0.0007505703 | f
378 | 3810 | 40 | 0.0012125460 | f
379 | 3820 | 40 | 0.0015003121 | f
380 | 3830 | 40 | 0.0015003121 | f
381 | 3840 | 40 | 0.0019676830 | f
382 | 3850 | 40 | 0.0019986490 | f
383 | 3860 | 40 | 0.0026327872 | f
384 | 3870 | 40 | 0.0132100396 | nadir
385 | 3880 | 40 | 0.0003014729 | f
386 | 3890 | 40 | 0.0007000497 | f
387 | 3900 | 40 | 0.0015319347 | f
388 | 3910 | 40 | 0.0017921951 | f
389 | 3920 | 40 | 0.0017921951 | f
390 | 3930 | 40 | 0.0022393860 | f
391 | 3940 | 40 | 0.0022719699 | f
392 | 3950 | 40 | 0.0030580100 | f
393 | 3960 | 40 | 0.000000E+00 | f
394 | 3970 | 40 | 0.0004499928 | f
395 | 3980 | 40 | 0.0004499928 | f
396 | 3990 | 40 | 0.0005820932 | f
397 | 4000 | 40 | 0.0015172891 | f
398 | 4010 | 40 | 0.0015172891 | f
399 | 4020 | 40 | 0.0013696883 | f
400 | 4030 | 40 | 0.0013696883 | f
401 | 4040 | 40 | 0.0020009629 | f
402 | 4050 | 40 | 0.0020009629 | f
403 | 4060 | 40 | 0.0020178476 | f
404 | 4070 | 40 | 0.0023154656 | f
405 | 4080 | 40 | 0.0025781792 | f
406 | 4090 | 40 | 0.000000E+00 | f
407 | 4100 | 40 | 0.0007638533 | f
408 | 4110 | 40 | 0.0285326087 | nadir
409 | 4120 | 40 | 0.0003736413 | f
410 | 4130 | 40 | 0.0008861701 | f
411 | 4140 | 40 | 0.0010854648 | f
412 | 4150 | 40 | 0.0010854648 | f
413 | 4160 | 40 | 0.0010854648 | f
414 | 4170 | 40 | 0.0016690487 | f
415 | 4180 | 40 | 0.0021193124 | f
416 | 4190 | 40 | 0.0022214292 | f
417 | 4200 | 40 | 0.0023204080 | f
418 | 4210 | 40 | 0.0030321199 | f
419 | 4220 | 40 | 0.0010818326 | f
420 | 4230 | 40 | 0.0014217426 | f
421 | 4240 | 40 | 0.0014217426 | f
422 | 4250 | 40 | 0.0018266239 | f
423 | 4260 | 40 | 0.0023245268 | f
424 | 4270 | 40 | 0.0028914241 | f
425 | 4280 | 40 | 0.0007839110 | f
426 | 4290 | 40 | 0.0007839110 | f
427 | 4300 | 40 | 0.0007839110 | f
428 | 4310 | 40 | 0.0009380045 | f
429 | 4320 | 40 | 0.0010040348 | f
430 | 4330 | 40 | 0.0023268932 | f
431 | 4340 | 40 | 0.0030104045 | f
432 | 4350 | 40 | 0.000000E+00 | f
433 | 4360 | 40 | 0.000000E+00 | f
434 | 4370 | 40 | 0.000000E+00 | f
435 | 4380 | 40 | 0.0002433649 | f
436 | 4390 | 40 | 0.0002433649 | f
437 | 4400 | 40 | 0.0007527534 | f
438 | 4410 | 40 | 0.0007527534 | f
439 | 4420 | 40 | 0.0009575380 | f
440 | 4430 | 40 | 0.0013003911 | f
441 | 4440 | 40 | 0.0013343585 | f
442 | 4450 | 40 | 0.0013343585 | f
443 | 4460 | 40 | 0.0027247956 | nadir
444 | 4470 | 40 | 0.0002043597 | f
445 | 4480 | 40 | 0.0002043597 | f
446 | 4490 | 40 | 0.0054794521 | nadir
447 | 4500 | 40 | 0.000000E+00 | f
448 | 4510 | 40 | 0.0002724153 | f
449 | 4520 | 40 | 0.0002724153 | f
450 | 4530 | 40 | 0.0002724153 | f
451 | 4540 | 40 | 0.0157630558 | nadir
452 | 4550 | 40 | 0.000000E+00 | f
453 | 4560 | 40 | 0.0003489110 | f
454 | 4570 | 40 | 0.0003489110 | f
455 | 4580 | 40 | 0.0006769637 | f
456 | 4590 | 40 | 0.0010035058 | f
457 | 4600 | 40 | 0.0019011139 | f
458 | 4610 | 40 | 0.0023117837 | f
459 | 4620 | 40 | 0.0023459835 | f
460 | 4630 | 40 | 0.0023801832 | f
461 | 4640 | 40 | 0.0023801832 | f
462 | 4650 | 40 | 0.0023801832 | f
463 | 4660 | 40 | 0.0023801832 | f
464 | 4670 | 40 | 0.0022433846 | f
465 | 4680 | 40 | 0.0022433846 | f
466 | 4690 | 40 | 0.0022775843 | f
467 | 4700 | 40 | 0.0024143832 | f
468 | 4710 | 40 | 0.0029028117 | f
469 | 4720 | 40 | 0.0094850949 | nadir
470 | 4730 | 40 | 0.0015386924 | f
471 | 4740 | 40 | 0.0016065350 | f
472 | 4750 | 40 | 0.0016307494 | f
473 | 4760 | 40 | 0.0018688742 | f
474 | 4770 | 40 | 0.0022868572 | f
475 | 4780 | 40 | 0.0029299565 | f
476 | 4790 | 40 | 0.000000E+00 | f
477 | 4800 | 40 | 0.000000E+00 | f
478 | 4810 | 40 | 0.000000E+00 | f
479 | 4820 | 40 | 0.0008668306 | f
480 | 4830 | 40 | 0.0008668306 | f
481 | 4840 | 40 | 0.0008668306 | f
482 | 4850 | 40 | 0.0011655384 | f
483 | 4860 | 40 | 0.0018100517 | f
484 | 4870 | 40 | 0.0018100517 | f
485 | 4880 | 40 | 0.0018100517 | f
486 | 4890 | 40 | 0.0018100517 | f
487 | 4900 | 40 | 0.0021821201 | f
488 | 4910 | 40 | 0.0054570259 | nadir
489 | 4920 | 40 | 0.000000E+00 | f
490 | 4930 | 40 | 0.0004058759 | f
491 | 4940 | 40 | 0.0006191377 | f
492 | 4950 | 40 | 0.0006191377 | f
493 | 4960 | 40 | 0.0018419639 | f
494 | 4970 | 40 | 0.0018419639 | f
495 | 4980 | 40 | 0.0028669034 | f
496 | 4990 | 40 | 0.000000E+00 | f
497 | 5000 | 40 | 0.0007420504 | f
498 | 5010 | 40 | 0.0014608306 | f
499 | 5020 | 40 | 0.0016415940 | f
500 | 5030 | 40 | 0.0020134866 | f
501 | 5040 | 40 | 0.0020816994 | f
502 | 5050 | 40 | 0.0023257430 | f
503 | 5060 | 40 | 0.0023939558 | f
504 | 5070 | 40 | 0.0031056945 | f
505 | 5080 | 40 | 0.0001364256 | f
506 | 5090 | 40 | 0.0008663032 | f
507 | 5100 | 40 | 0.0008663032 | f
508 | 5110 | 40 | 0.0008663032 | f
509 | 5120 | 40 | 0.0009687233 | f
510 | 5130 | 40 | 0.0009687233 | f
511 | 5140 | 40 | 0.0009687233 | f
512 | 5150 | 40 | 0.0014624199 | f
513 | 5160 | 40 | 0.0014624199 | f
514 | 5170 | 40 | 0.0014624199 | f
515 | 5180 | 40 | 0.0014624199 | f
516 | 5190 | 40 | 0.0020791360 | f
517 | 5200 | 40 | 0.0020791360 | f
518 | 5210 | 40 | 0.0021132424 | f
519 | 5220 | 40 | 0.0021132424 | f
520 | 5230 | 40 | 0.0021132424 | f
521 | 5240 | 40 | 0.0021132424 | f
#print(res.history[0].pop.get("F"))
11.5. Analysis of Results#
import plotly.express as px
fig = px.scatter(res.F, x=0, y=1, labels={'0': 'Efficiency', '1': 'Volume'},
title="In Efficiency vs Volume",
width=800, height=800)
fig.update_traces(marker=dict(size=8,
line=dict(width=1,
color='DarkSlateGrey')),
selector=dict(mode='markers'))
fig.show()
# Making a animation of evolution
import pandas as pd
import plotly.express as px
obj1 = []
obj2 = []
calls = []
for r in res.history:
objs = r.pop.get("F")
obj1.extend(objs[:, 0])
obj2.extend(objs[:, 1])
calls.extend([r.n_gen]*len(objs))
df = pd.DataFrame(data = {"InEfficiency": obj1, "Volume": obj2, "n_gen": calls})
obj_fig = px.scatter(df, x="InEfficiency", y="Volume",
animation_frame="n_gen", color="n_gen",
range_x=[0., 0.6], range_y=[0. , 400.],
hover_data = df.columns,
width = 800, height = 800)
obj_fig.update(layout_coloraxis_showscale=False)
obj_fig.layout.updatemenus[0].buttons[0].args[1]["frame"]["duration"] = 10
obj_fig.update_layout(transition = {'duration': 0.001})
obj_fig.show()
# Computing hyper volume
## Tracking hypervolume
from pymoo.indicators.hv import HV
import plotly.graph_objs as go
ref_point = np.array([0.8, 350.0])
hv_list = []
for gen in res.history:
hv = HV(ref_point)(gen.pop.get("F"))
hv_list.append(hv)
fig = go.Figure()
fig.add_trace(go.Scatter(x=np.arange(1, len(hv_list)+1), y=hv_list, mode='lines+markers'))
fig.update_layout(title='Hypervolume Tracking', xaxis_title='Generation', yaxis_title='Hypervolume')
fig.show()
print ("Hypervolume at the end of the optimization: ", hv_list[-1])
Hypervolume at the end of the optimization: 180.04826266450968
# A parallel plot of design adn objective space
import plotly.graph_objects as go
X = res.pop.get("X")
F = res.pop.get("F")
fig = go.Figure(data=go.Parcoords(
line=dict(color=F[:, 0],
colorscale='Viridis',
showscale=True,
reversescale=True),
dimensions = [dict(range = [xl[index], xu[index]], label = f'X{index}', values=X[:, index])
for index in range(len(xl))
]
))
fig.update_layout(title = "Parallel plot of design space and objective space",
template='plotly_dark')
fig.show()
fig = go.Figure(data=go.Parcoords(
line=dict(color=F[:, 1],
colorscale='Viridis',
showscale=True,
reversescale=True),
dimensions = [dict(range = [xl[index], xu[index]], label = f'X{index}', values=X[:, index])
for index in range(len(xl))
]
))
fig.update_layout(title = "Parallel plot of design space and objective space",
template='plotly_dark')
fig.show()
11.6. Exercise#
Can you plot the hypervolume metric to see if the convergence is reached
Can you implement a termination criterion
Plot the optimal configuration of the tracker corresponding to that point
Do analysis of convergence
Visualize the point with a radar or petal diagram, following https://pymoo.org/visualization/index.html